If you've ever wanted to create your own NPM package, share it with the world, and have others benefit from it, you're in the right place! In this article, we'll walk through the process of creating an NPM package from scratch, publishing it to the NPM registry, and making it publicly available for anyone to use.
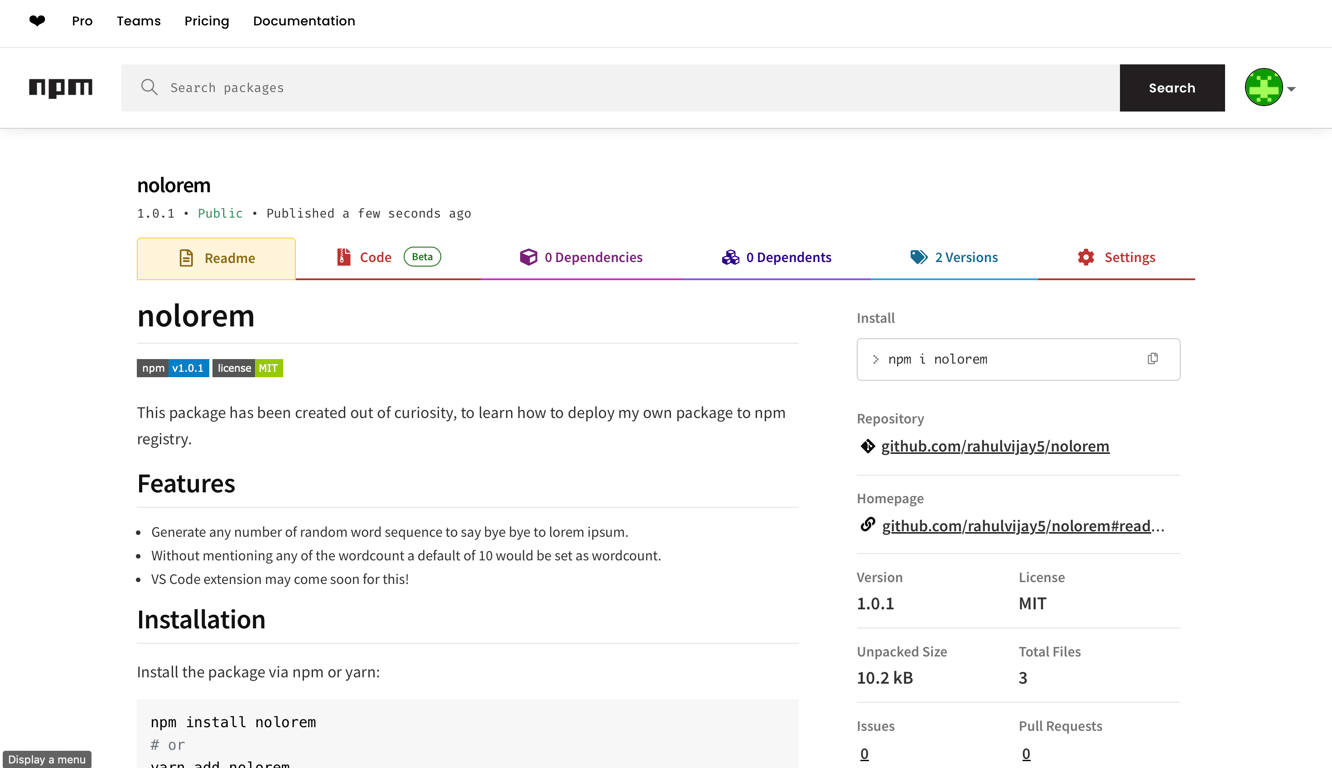
In this example, we'll be creating a package called nolorem, a utility designed to replace Lorem Ipsum text generation with more meaningful placeholder text. This package will allow users to pass a word count into a function and generate custom text, which can help developers avoid overused placeholder text.
Let's dive in and break down the steps to create your own NPM package.
1. Check for Package Name Availability
Before you start coding, check if your chosen package name is already taken on NPM. This is critical because package names must be unique across the entire registry. To check, you can search for your package name on [NPM's website](https://www.npmjs.com/) or simply run the following command in your terminal:
npm search nolorem
If the name is available, you're good to go. If not, you’ll need to come up with a different name. Once you have an available name, we can proceed to the next step.
2. Create a New Folder for Your Package
Start by creating a new directory on your computer where you'll store your package:
mkdir nolorem
cd nolorem
Inside this folder, we'll initialise our NPM package and create the necessary files.
3. Initialize Your Package
Next, run the following command to initialise your package:
npm init
This will prompt you to fill in some basic information about your package (e.g., name, version, description). You can either press Enter to accept the default options or fill them in manually.
For example:
- name: nolorem
- version: 1.0.0
- description: A package to generate meaningful placeholder text.
- entry point: index.js (this is where your main code will reside)
Once you've filled in the details, this will create a package.json
file in your project directory, which NPM uses to manage your package.
4. Create the Core Functionality (index.js)
Now it's time to write the core functionality of your package. Let's create an index.js
file that will contain a simple function for generating meaningful placeholder text based on a word count.
Create a file named index.js
in the root of your project folder and add the following code:
//index.js
function nolorem(wordCount) {
const words = ['apple', 'banana', 'orange', 'grape', 'pineapple', 'kiwi', 'mango', 'pear', 'peach', 'plum'];
let text = '';
for (let i = 0; i < wordCount; i++) {
text += words[i % words.length] + ' ';
}
return text.trim();
}
module.exports = nolorem;
This function generates a simple string of words based on the count you pass in. You can expand this functionality as needed, but for now, it works as a placeholder for the package.
5. Test Your Package Locally
Before publishing your package, it's a good idea to test it locally. To do so, you can use npm link
to create a symlink to your package and use it in another project.
Step 1: Link Your Package
In the root folder of your package (where index.js
is), run:
npm link
This will create a global symlink to your package.
Step 2: Create a Test Folder
Create a new folder called test
and add a script.js
file inside it:
mkdir test
cd test
touch script.js
Inside script.js
, add the following code to test your package:
const nolorem = require('nolorem');
console.log(nolorem(10));
Step 3: Link the Package in the Test Folder
Now, navigate to the test
folder and run:
npm link nolorem
This will link your local nolorem
package to the test script.
Step 4: Run the Test
Now, you can run the script to test if your package works as expected:
node script.js
If everything works correctly, you should see a string of words printed to the console.
6. Publish Your Package to NPM
Once your package is working locally, it's time to make it available to the public. But first, you'll need to create an account on [npmjs.com](https://npmjs.com/).
Step 1: Log in to NPM
Run the following command to log in to your NPM account:
npm login
This will prompt you to enter your NPM username, password, and email address.
Step 2: Publish Your Package
After logging in, run the following command to publish your package:
npm publish
This will upload your package to the NPM registry, making it publicly available. Anyone can now install and use your package by running:
npm install nolorem
7. Update Your Package
In the future, if you need to update your package, it's important to follow Semantic Versioning (SemVer). SemVer uses a versioning format like 1.2.3
, where:
- The leftmost digit (major version) represents significant changes or breaking changes.
- The middle digit (minor version) represents backward-compatible changes.
- The rightmost digit (patch version) represents bug fixes.
Step 1: Bump Version
If you made a bug fix, you can bump the patch version with the following command:
npm version patch
For a new feature or change, you might bump the minor version:
npm version minor
Or, for a breaking change:
npm version major
Step 2: Publish the New Version
After updating the version, you can run npm publish
again to push the new version to the registry.
8. Add a README.md File
It’s important to add a README.md
file to your package to help users understand how to use it. In the root of your project, create a file called README.md
and add instructions for installation and usage like: Title Name, Description, Usage and etc.
Conclusion
Congratulations! You've successfully created and published your first NPM package. You've learned how to:
- Check for available package names
- Initialize a new NPM project
- Write core functionality for your package
- Test the package locally
- Publish your package to the NPM registry
- Update your package following Semantic Versioning
If you want you may see my package: Here
Also this article: link from freecodecamp was supernal for me to learn this on my own too.
Now your package is live, and others can easily install and use it! Don't forget to maintain your package by fixing bugs, adding features, and keeping it up-to-date on GitHub.
Happy coding!